How can I convert a number to a currency format in JavaScript with commas?Alex K![alex k profile pic]()
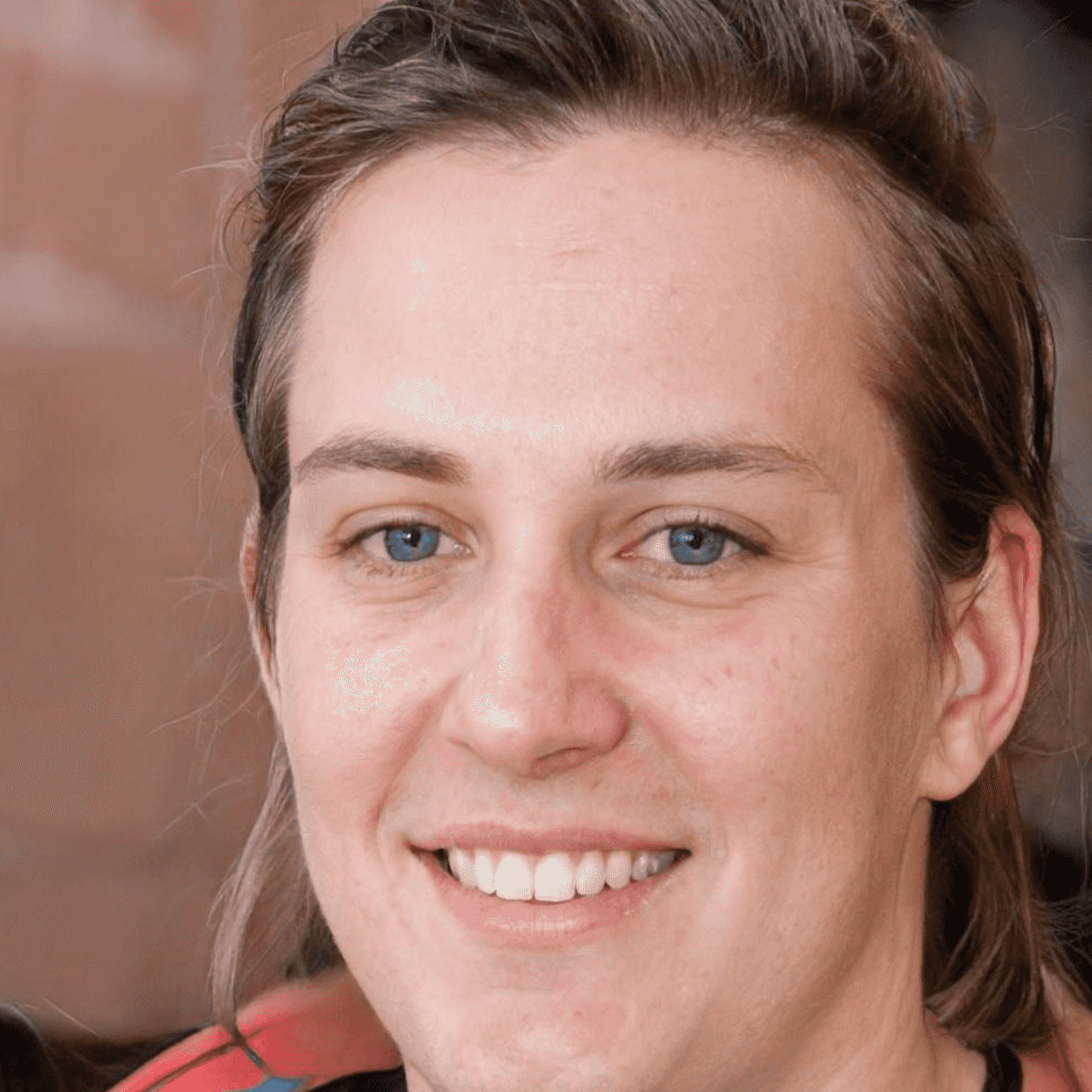
To convert a number to a currency format in JavaScript with commas, you can use a combination of JavaScript's built-in methods and some formatting logic. Here's a step-by-step approach: 1. Convert the number to a string:
1 2 3 4
const number = 1234567.89; const numberString = number.toString();
2. Split the number string into an integer part and a decimal part:
1 2 3 4 5
const parts = numberString.split('.'); const integerPart = parts[0]; const decimalPart = parts[1] || '';
3. Insert commas in the integer part:
1 2 3
const formattedInteger = integerPart.replace(/\B(?=(\d{3})+(?!\d))/g, ',');
4. Combine the formatted integer part with the decimal part:
1 2 3
const formattedNumber = decimalPart ? `${formattedInteger}.${decimalPart}` : formattedInteger;
5. Add a currency symbol, if desired:
1 2 3 4
const currencySymbol = '$'; const formattedCurrency = `${currencySymbol}${formattedNumber}`;
NowformattedCurrency
will contain the number formatted as a currency with commas.
Here's an example that puts it all together:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
function formatCurrency(number, currencySymbol = '$') { const numberString = number.toString(); const parts = numberString.split('.'); const integerPart = parts[0]; const decimalPart = parts[1] || ''; const formattedInteger = integerPart.replace(/\B(?=(\d{3})+(?!\d))/g, ','); const formattedNumber = decimalPart ? `${formattedInteger}.${decimalPart}` : formattedInteger; const formattedCurrency = `${currencySymbol}${formattedNumber}`; return formattedCurrency; } const amount = 1234567.89; const formattedAmount = formatCurrency(amount); console.log(formattedAmount); // Output: $1,234,567.89
You can customize the currency symbol by passing a different value for thecurrencySymbol
parameter when calling theformatCurrency
function.
Similar Questions
How can I convert a number to a currency format in JavaScript?
How can I convert a string to a number in JavaScript?
How can I convert a number to a Roman numeral in JavaScript?
How can I convert a number to Roman numerals in JavaScript?
How can I convert a number to a string with leading zeros in JavaScript?
How can I convert a number to a string with leading zeros in JavaScript?
How can I convert a number to a binary string in JavaScript?
How can I convert an array to a set in JavaScript?
How can I convert an array to a set in JavaScript?
How can I convert a string to a number with a specific base in JavaScript?
How do I convert a number to a string in JavaScript?
How can I convert a string to a date object in JavaScript with a specific format?
How can I convert a JavaScript array to a string with a custom separator?
How can I convert a string to a floating-point number in JavaScript?
How can I convert an object to a set in JavaScript?
How can I convert an array to a comma-separated string in JavaScript?
How can I convert an array to a comma-separated string in JavaScript?
How can I convert a number to a specific number of decimal places in JavaScript?
How can I convert a JavaScript array to a CSV string with custom column headers?
How can I convert a number to a string with a specific number of decimal places in JavaScript?